Harvey, a .net chat server built with RabbitMQ
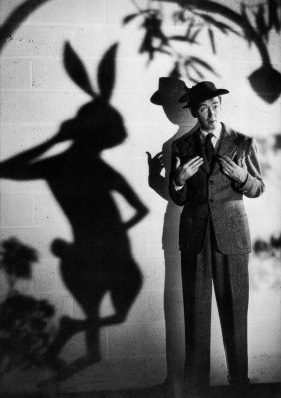
I've turned into a rabbid RabbitMQ fan in the last week or two, though so far I've only scratched the surface of what this thing does.
Below I'm going to walk through the code for a chat service, built with .net, that uses RabbitMQ for sending and receiving messages. But first a short discussion of Message Queues, RabbitMQ, and how to get this rabbit up and running.
A lengthy discussion is out of scope for this bus ride, but basically:
A message-queue is a piece of middleware for asynchronous communication. (System A sends messages to System B).
MQ's can be optimized for performance, reliability, scalability or any other '*ility' you can think to mention.
There's lots of them, they make different trade offs. Originally they were expensive proprietary technologies (e.g IBM's MQ-Series) - but along with the rise of standards in this area there have arisen various compelling open source offerings.
RabbitMQ is built on Erlang. I don't want to digress into sounding like one of those Erlang-douchebags, but Erlang is a good match for an MQ.
Erlang's initial purpose was to create telecommunications software that was (a) super reliable and (b) hot-swappable. That's a perfect fit for MQ software. It can spin up extra processes without all the heavy lifting of using extra threads, so where a normal OS thread allocates a few megs of memory, Erlang gets away with a few bytes. Extraordinary stuff.
Having said that, the biggest problem with RabbitMQ is that it's built on Erlang. Thus, to install it on your Enterprise-controlled Servers at BigCo you'll need to get Corporate IT's permission to install yet another VM/Platform. Good luck sweet talking those guys. They do *love* to kick up a fuss.
Up and running with RabbitMQ in Under 3 minutes
Everything I'm going to cover in this section is covered in part 1 of Derek Greer's RabbitMQ for windows series. So I'll go extra quick.
To setup a host server for your chatting you'll need to...
- Install erlang: http://www.erlang.org/download.html
- Set the
ERLANG_HOME
environment variable to point to the erlang folder under program files. e.g.C:\Program Files\erl5.9.2
- Install rabbitMQ: http://www.rabbitmq.com/download.html
- Enable the rabbitmq management plugin. from an elevated cmd prompt:
Go to rabbit'ssbin
folder, e.g.%programfiles%\RabbitMQ Server\rabbitmq_server-2.8.7\sbin
, and run:
rabbitmq-plugins.bat enable rabbitmq_management
- To activate the management plugin, stop, install and start the rabbitmq service:
rabbitmq-service.bat stop
rabbitmq-service.bat install
rabbitmq-service.bat start
- Finally, visit http://localhost:55672/mgmt/ and see that your rabbitMQ instance is alive.
It's *that* simple.
Worlds easier than most other installs. Much easier than installing a database, or keeping Adobe Reader up to date.
The only other thing you need do to become a certified .net RabbitMQ developer is use nuget to add a reference to the RabbitMQ.client package.
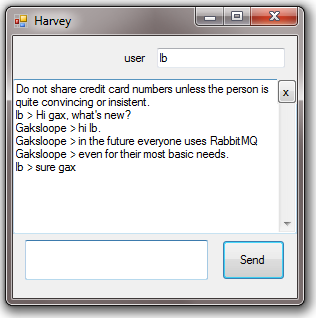
Introducing Harvey (the simple .net chat client)
Once your rabbitMQ service is up and running, every one on your network can grab Harvey.exe and join in one colossal chat room for all their communication purposes. Every message is delivered to every listener.
The architecture is simple. When you run Harvey.exe it creates two channels, one for sending, one for receiving. The send channel is connected to a fan-out exchange on the server. Each Harvey client also creates its own queue on the server (identified by a guid), which is bound to the afore mentioned fan-out exchange. Thus, when any client sends a message, every client receives it.
Let's step through it.
Set up a channel to the fanout exchange
(Just let it wash over you, this will all make sense by the end)
In form_load
we setup everything we need for sending messages. We need a channel to the exchange. The exchange is of type 'fanout' meaning it will send all messages to all queues that are bound to it.
When we 'declare' the exchange, the exchange will be created on the server if it doesn't already exist. Otherwise we will use the existing exchange that has already been declared for us.
In form_load
:
var connectionFactory = new ConnectionFactory { HostName = "localhost", Port = 5672, UserName = "guest", Password = "guest", VirtualHost = "/" }; connection = connectionFactory.CreateConnection(); channelSend = connection.CreateModel(); channelSend.ExchangeDeclare(exchangeName, ExchangeType.Fanout, false, true, null);
Sending a message
Assuming we have a textbox (txtMessage
) for entering the message we want to post, here's what happens when we click send:
string input = txtUserName.Text + " > " + txtMessage.Text; byte[] message = Encoding.UTF8.GetBytes(input); channelSend.BasicPublish(exchangeName, "", null, message); txtMessage.Text = string.Empty; txtMessage.Focus();
That was nice, but we probably want to receives messages back as well -- a chat is not just one way.
Set up a channel to your own queue, for receiving.
We declare a queue, a brand new queue that no one has declared before, and bind it to the fanout exchange.
So messages sent to that exchange will go to this queue, on the server. And we've got a channel to the queue.
(This bit also happens in form_load
)
channelReceive = connection.CreateModel(); channelReceive.QueueDeclare(clientId, false, false, true, null); channelReceive.QueueBind(clientId, exchangeName, "");
Receiving a message...
The very next thing we do in form_load
, is start a thread for listening to messages on that channel:
receivingThread = new Thread(() => channelReceive.StartConsume(clientId, MessageHandler)); receivingThread.Start();
(Note, forgetting to call .Start() cost me more debugging time than anything else in this whole learning experience)
The following 'StartConsume
' extension method was lifted from one of Derek Greer's RabbitMQ articles:
We block the thread waiting for a Dequeue
to happen.
public static void StartConsume(this IModel channel, string queueName, Action<IModel, DefaultBasicConsumer, BasicDeliverEventArgs> callback) { QueueingBasicConsumer consumer = new QueueingBasicConsumer(channel); channel.BasicConsume(queueName, true, consumer); while (true) { try { var eventArgs = (BasicDeliverEventArgs)consumer.Queue.Dequeue(); callback(channel, consumer, eventArgs); } catch (EndOfStreamException) { // The consumer was cancelled, the model closed, or the connection went away. break; } } }
And the 'MessageHandler
' delegate, above is as follows:
public void MessageHandler(IModel channel, DefaultBasicConsumer consumer, BasicDeliverEventArgs eventArgs) { string message = Encoding.UTF8.GetString(eventArgs.Body) + "\r\n"; txtConversation.InvokeIfRequired(() => { txtConversation.Text += message; txtConversation.ScrollToEnd(); }); }
InvokeIfRequired
is just a useful winforms extension method for hopping from a background thread onto the gui thread, taken from this stackoverflow question, and implemented as follows:
public static void InvokeIfRequired(this Control control, MethodInvoker action) { if (control.InvokeRequired) { control.Invoke(action); } else { action(); } }
Further reading:
This guy used a similar architecture to what i went with. It's just the simplest architecture imaginable, and he handled 2000 messages a second from a very minimal piece of hardware.
Simon Dixon's article - Getting Started With RabbitMQ in .net
Mike Hadlow has written 'an easy to use .net api for RabbitMQ' called EasyNetQ. One to watch.
As recommended above, Derek Greer has an Excellent Series on RabbitMQ for Windows
Further links to .net development with RabbitMQ
Next → ← PreviousMy book "Choose Your First Product" is available now.
It gives you 4 easy steps to find and validate a humble product idea.