hgs: Manage Lots of Mercurial Projects Simultaneously
hgs
is a simple set of powershell functions that let you find and manage multiple mercurial projects -- simultaneously.
Background
A friend of mine creates way too many mercurial repositories. Where other people would have multiple projects in one solution, he has numerous individual projects each in their own repository (which are sometimes shared back into each other via nuget packages).
The result is a very explicit separation of concerns, but also a *lot* of repositories. Just trying to get all of his changes involves pulling from many different repositories.
This gave me the motivation I need to come up with a simpler way to manage multiple repositories.
At first I decided to make a windows app that showed all your repositories at once, and let you select many of them to run a command against simultaneously. Here's the basic layout I was thinking of:
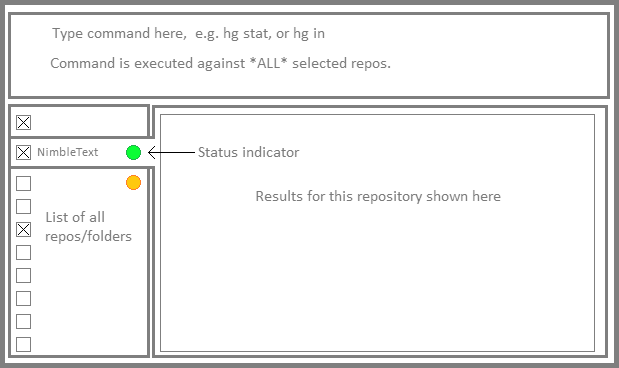
The clever part is that the result of any command would be parsed by a series of regular expressions that are used for determining the status of each repository, relative to that command. Thus you can see each repo light up as green, when your multiple pulls succeed, or red when your multiple updates fail, etc. And a lot of effort would be spent on having first-class keyboard support, so your mouse is free to gather dust.
To get this working would've taken many bus-rides to do the way I wanted, and a lot longer to maintain and improve it.
Fuck that.
Instead I wrote a page of powershell, and got the same result.
Introducing hgs
-- the plural of hg.
To get started, grab the .ps1 file above. Then, from powershell, dot-source the file to install the four functions. (Oh, read it first if you must.)
hgs
is composed of four simple functions. Here it is in its entirety:
# function hgs_update -- updates/refreshes "hgs.txt" function hgs_update() { dir . .hg -recurse | % { $_.Parent.FullName } > $env:localappdata"\hgs.txt" } # function hgs_ls -- list the contents of hgs.txt function hgs_ls () { get-content $env:localappdata"\hgs.txt" } #function hgs -- runs hg commands against all folders in hgs # usage: hgs {commands}, examples: hgs stat, hgs in, hgs fetch function hgs() { $a = $args; $color = $host.UI.RawUI.ForegroundColor; type $env:localappdata"\hgs.txt" | % { pushd $_; $host.UI.RawUI.ForegroundColor ="White"; "Results for: " + $_ + ":"; $host.UI.RawUI.ForegroundColor =$color ; hg $a; popd } } #function hgs_measure # runs hg commands against all folders in hgs, return length of response (in rows) # usage: hgs_measure {commands} # examples: hgs_measure stat, hgs_measure in, hgs_measure fetch function hgs_measure() { $a = $args; $color = $host.UI.RawUI.ForegroundColor; type $env:localappdata"\hgs.txt" | % { pushd $_; $host.UI.RawUI.ForegroundColor ="Red"; $output = hg $a | out-string; $host.UI.RawUI.ForegroundColor ="White"; "" + ($output.split("`n").length) + " lines: " + $_ ; $host.UI.RawUI.ForegroundColor =$color ; popd } }
All four functions are concerned with a file called 'hgs.txt' a file containing a list of all your mercurial repositories.
hgs_update
To update the hgs.txt file, call the function 'hgs_update
'.
This function pipes the name of every folder which contains a folder named '.hg' into hgs.txt (in other words, it searches for all your mercurial repos).
hgs_ls
The second function, hgs_ls
gives you a peak at the content of hgs.txt (i.e. it shows you all the mercurial repos that were found).
hgs
The third function, hgs
lets you run a hg
command against all the repositories at once.
For example you might try:
hgs stat # check the status of all your repositories hgs in # show new changesets for all your repositories hgs diff # diff all your repositories hgs pull -u # pull from all remote repositories and update if changes were found hgs fetch # pull changes from all remote repositories, merge new changes if needed. # (requires the unloved fetch extension)
I provide those examples because they are all things that you might do 'en masse'.
Other commands like revert
, forget
and definitely push
should be done on a more careful case-by-case basis.
hgs_measure
Finally, hgs_measure
, (like the hgs
command), lets you run a hg
command against all the repositories at once, but instead of showing you the results, it just tells you how many lines of text came back as a result. This is a way to get a quick overview of all your repositories without scrolling through screens and screens of information.
And powershell's wonderful code completion feature means that you only have to remember hgs
(the plural of hg) and you can tab through all four functions.
Best of luck.
Next → ← PreviousMy book "Choose Your First Product" is available now.
It gives you 4 easy steps to find and validate a humble product idea.