Custom Errors in ASP.Net MVC: It couldn't be simpler, right?
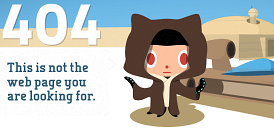
See til.secretgeek: MVC custom errors for some serious guidance. The lengthy article below is devilish trickery from a time when I was in a dark place.
Cool looking 404 pages are the new hotness. Github has an amazing parallaxing 404 page that allegedly cost more than any other feature on their site.
For a lot of sites, the 404 page is the most visited page, so it's worth getting it right.
The website for my new product, NimbleText, uses asp.net mvc. A framework I really enjoy. The gu wrote it on a plane. Before takeoff.
One of the more voodoo aspects of getting NimbleText.com into production was setting up a succesful custom 404 page. Here's what I came up with: check it out.
Some of the articles out there that cover custom errors in asp.net MVC seemed to be a little bit confused about exactly what is going on, many are out of date or incomplete and some are downright misleading.
So once and for all I want to give a definitive guide to error handling in asp.net MVC.
Here we are. Just eleven simple steps to follow for amazing results.
First, map a catch-all route in global.asax
, at the end of your other routes. E.g.
routes.MapRoute(
"404-PageNotFound",
"{*url}",
new { controller = "StaticContent", action = "PageNotFound" }
);
Second, create an Error Controller, like this:
public class ErrorController : Controller
{
[AcceptVerbs(HttpVerbs.Get)]
public ViewResult Unknown()
{
Response.StatusCode = (int)HttpStatusCode.InternalServerError;
return View("Unknown");
}
[AcceptVerbs(HttpVerbs.Get)]
public ViewResult NotFound(string path)
{
Response.StatusCode = (int)HttpStatusCode.NotFound;
return View("NotFound", path);
}
}
Third and fourth -- create custom views to handle the Unknown and NotFound actions above.
Fifth, create a page called Custom404.htm and add it to the root of your application. Use it to display a helpful, edgy and hopefully cool message. But don't be too edgy.
Sixth, add this to web.config, inside the system.web
node:
<customErrors mode="RemoteOnly" defaultRedirect="GenericErrorPage.htm">
<error statusCode="404" redirect="/Custom404.htm" />
</customErrors>
Seventh, add a httpErrors element inside the system.webServer
node:
<httpErrors>
<error statusCode="404" subStatusCode="-1" path="/custom404.htm" responseMode="Redirect" />
</httpErrors>
Eighth, inside your existing controllers, if there is no data to serve (e.g. if someone asks for a UserId that doesn't exist) then use this snippet of code:
throw new HttpException(404);
Ninth, inside Global.asax, look for the RegisterGlobalFilters
method (it will be called during Application_Start
), and add another global filter, to handle any specific exceptions in your code, like this:
filters.Add(new HandleErrorAttribute
{
ExceptionType = typeof(FileNotFoundException),
View = "Custom404", // Custom404.cshtml is a view in the Shared folder.
Order = 2
});
Tenth, add a view called Custom404.cshtml
under views/shared.
And, eleventh, take care to handle the global application error event, capturing and logging any errors, before redirecting to the views you're after:
protected void Application_Error(object sender, EventArgs e)
{
Exception exception = Server.GetLastError();
Response.Clear();
HttpException httpException = exception as HttpException;
if (httpException != null)
{
string action;
switch (httpException.GetHttpCode())
{
case 404:
// page not found
action = "HttpError404";
break;
case 500:
// server error
action = "Internal500";
break;
default:
action = "General";
break;
}
// clear error on server
Server.ClearError();
Response.Redirect(String.Format("~/Error/{0}/?message={1}", action, exception.Message));
}
}
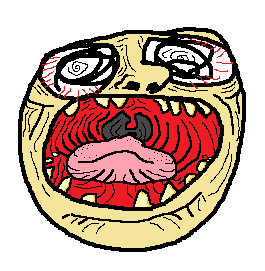
Good.
Now your website is well and truly borked.
Every request will bounce around your application like a demented pinball tripping on acid.
When an error happens, a bunch of different code modules will go to war.
The victorious code module will tear out the entrails of all those who oppose it, and throw them in the visitor's face.
You'll get a different error handler depending on what version of IIS you have, what version of MVC you're using, whether you've deployed in debug or release, whether you're visiting locally or remotely, whether it's sunny or raining.
Your server will be reduced to a pile of smouldering rubble from which reboot is impossible.
But if you want to get truly weird, try setting up a custom 403 page. That's about three times as odd.
Or, switch to Dos on Dope*. Life will be simple again.
Sorry I didn't really have time to write a definitive guide to this stuff. Error handling in MVC is crazy man, I'm busy fighting a clandestine war against hackers and crackers and bots and beuracrats: I can't be documenting that kind of mystery wrapped in an enigma.
If someone does write a complete guide to error handling in MVC, that includes the meaning and interaction behind each of these snippets, plus how the interplay between IIS, asp.net and MVC truly works, then I will happily update this article to include a link to it, right up top.
* Don't switch to Dos On Dope.
See til.secretgeek: MVC custom errors for some serious guidance. The article above is devilish trickery from a time when I was in a dark place.
Next → ← PreviousMy book "Choose Your First Product" is available now.
It gives you 4 easy steps to find and validate a humble product idea.