Further proof that testing is for wimps and bad programmers
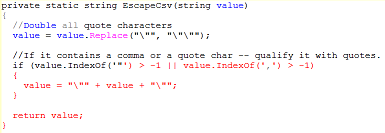
Couple of days ago I wrote a C# function for a colleague and emailed it to him.
This is the function:
private static string EscapeCsv(string value)
{
//Double all quote characters
value = value.Replace("\"", "\"\"");
//If it contains a comma or a quote char -- qualify it with quotes.
if (value.IndexOf('"') > -1 || value.IndexOf(',') > -1)
{
value = "\"" + value + "\"";
}
return value;
}
The syntax highlighting looks very strange, because I wrote it in sql server management studio. It just happened that the only text editor i had open at the time was SQL server, so that's what i used.
(Real developers don't use any particular editor -- they just use whatever's open at the time. Even the act of opening notepad is too cumbersome for the ubergeek.)
After I emailed it to my colleague I had a moment of weakness, when I suffered the tiniest smidgen of self doubt.
"Perhaps I should've tested the code in some way. Or -- at least -- compiled it?"
Scott Bellware and the cool alt.net kids are always banging on about this sort of stuff, so I wrote an awesome console app to test it.
namespace tests_are_for_wusses
{
class Program
{
static void Main(string[] args)
{
System.Console.WriteLine(EscapeCsv("fred") == "fred");
System.Console.WriteLine(EscapeCsv("fre,d") == "\"fre,d\"");
System.Console.WriteLine(EscapeCsv("fre\"d") == "\"fre\"\"d\"");
System.Console.ReadLine();
}
private static string EscapeCsv(string value)
{
//Double all quote characters
value = value.Replace("\"", "\"\"");
//If it contains a comma or a quote char -- qualify it with quotes.
if (value.IndexOf('"') > -1 || value.IndexOf(',') > -1)
{
value = "\"" + value + "\"";
}
return value;
}
}
}
Naturally, the awesome code passed my awesome tests first go.
Thus I have once again shown that testing is a waste of time if you are awesome like me.
And the so-called cool kids from alt.net are just bad programmers.
Thank you.
Wait a second... I still feel I'm missing the point. Care to enlighten me?
(By the way -- pretty much every sentence in this post was sarcastic... while the story is true, my real interpretation is that i just got lucky this time. There's definitely some bugs still hidden even in a simple function like this)
Next → ← PreviousMy book "Choose Your First Product" is available now.
It gives you 4 easy steps to find and validate a humble product idea.